Working with Long numbers in mongoose
When you need to handle large numbers you need `mongoose-long` npm module. Here is how you can do it.
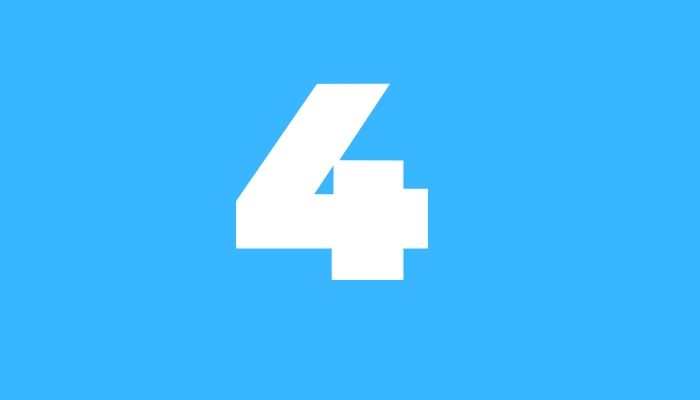
For most of the cases, the Number
SchemaType works well for handling Integers and Floats in Mongoose, but when you need to handle large numbers you need the mongoose-long
npm module. Here is how you can do it.
Suppose You have a mongoose schema Part and you have a field referenceId
which stores a very big integer (e.g. 100000000000000005).
Get Started
Install the package
npm i mongoose-long
Here is the mongoose schema.
const mongoose = require("mongoose");
let Schema = mongoose.Schema;
require("mongoose-long")(mongoose);
mongoose.connect("mongodb://localhost/appsyoda", { useNewUrlParser: true });
const db = mongoose.connection;
db.on("error", console.error.bind(console, "connection error:"));
db.once("open", function() {
// we're connected!
console.log("connected");
});
const SchemaTypes = mongoose.Schema.Types;
const partSchema = new Schema({ referenceId: SchemaTypes.Long });
const Part = db.model("Part", partSchema);
The mongoose-long
the package gives you a new Schema type which is mongoose.Schema.Types.Long
(it is not available by default in mongoose for unknown reason) and this is the schema type that allows you the use of big integers in mongoose.
Increment a Big Integer
Suppose you wanted to increment the value of referenceId
property a given part object. Here is what you need to do.
const Long = mongoose.Types.Long;
console.log("referenceId before " + part.referenceId);
part.referenceId = part.referenceId.add(Long.fromString("1"));
part.save();
console.log("referenceId after " + part.referenceId);
Here is the output you’ll get
$ node long-numbers-mongoose.js
referenceId before 100000000000000005
referenceId after100000000000000006
I hope you get the idea.
Multiply a Big Integer
Suppose you want to multiply the value inside referenceId to some other value. You need to use the multiply function. Check the code snippet below.
const part = new Part({ referenceId: "100000000005" });
const Long = mongoose.Types.Long;
console.log("referenceid before " + part.referenceId);
part.referenceId = part.referenceId.multiply(Long.fromString("100"));
part.save();
console.log("referenceid after " + part.referenceId);
Here is the output you will get
Output:
$ node long-numbers-mongoose.js
referenceid before 100000000005
referenceid after 10000000000500
Similarly, for division, you can use the div
function.
Comparing Long Integers in Mongoose
For comparing large numbers in mongoose, you can use various functions like compare
, greaterThanOrEqual
, greaterThan
, lessThanOrEqual
, lessThan
, notEquals
, equals
Here is the sample code snippet.
const part1 = new Part({ referenceId: "5700000000700" });
const part2 = new Part({ referenceId: "5678998891993" });
const part3 = new Part({ referenceId: "473266283872387" });
const part4 = new Part({ referenceId: "56683832733232" });
const Long = mongoose.Types.Long;
console.log("compare " + part1.referenceId.compare(part2.referenceId));
console.log("greaterThanOrEqual " + part3.referenceId.greaterThanOrEqual(part4.referenceId));
console.log("comgreaterThan " + part1.referenceId.greaterThan(part4.referenceId));
console.log("lessThanOrEqual " + part3.referenceId.lessThanOrEqual(part2.referenceId));
console.log("lessThan " + part2.referenceId.lessThan(part4.referenceId));
console.log("notEquals " + part1.referenceId.notEquals(part1.referenceId));
console.log("equals " + part1.referenceId.equals(part1.referenceId));
Here is the output you will get:
$ node long-numbers-mongoose.js
compare 1
greaterThanOrEqual true
comgreaterThan false
lessThanOrEqual false
lessThan true
notEquals false
equals true
Conclusion
By default, mongoose doesn’t allow you to deal with large numbers, but once you use the npm package mongoose-long
, you can deal with large numbers, store them in MongoDB. You can check the list of all the functions available with Long here
Write your doubts/queries in the comments section.
WRITTEN BY
Mohit Sehgal
Want to positively impact the world. Full Stack Developer, MEVN Stack (MongoDB, Express.js, Vue.js, Node.js)